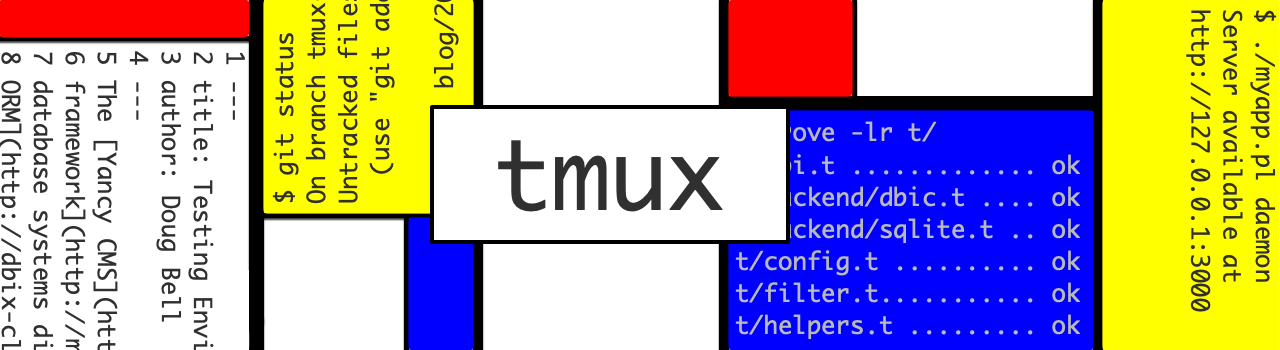
The Yancy CMS for the Mojolicious web framework currently supports three different database systems directly (and even more through the DBIx::Class ORM). As a result, when doing development, I need to have two database daemons running locally, a bunch of different environment variables to tell the tests where those databases are, and a web daemon to test the front-end.
Setting up these daemons is a pain, but I also do not want to run them all the time (to save on my laptop's battery). To me, it's easier to run a database daemon for a specific project than to try to manage all the databases I might need. But that means that every time I want to do some work on Yancy, I need to start up a bunch of things.
Since I do all my development in a terminal window, the Tmux terminal multiplexer has become an extremely useful tool. Using a shell script and Tmux, I can run a single command to set up all the databases, environment variables, and all the tabs I need to get to work quickly.